Swift Coding Made Easy
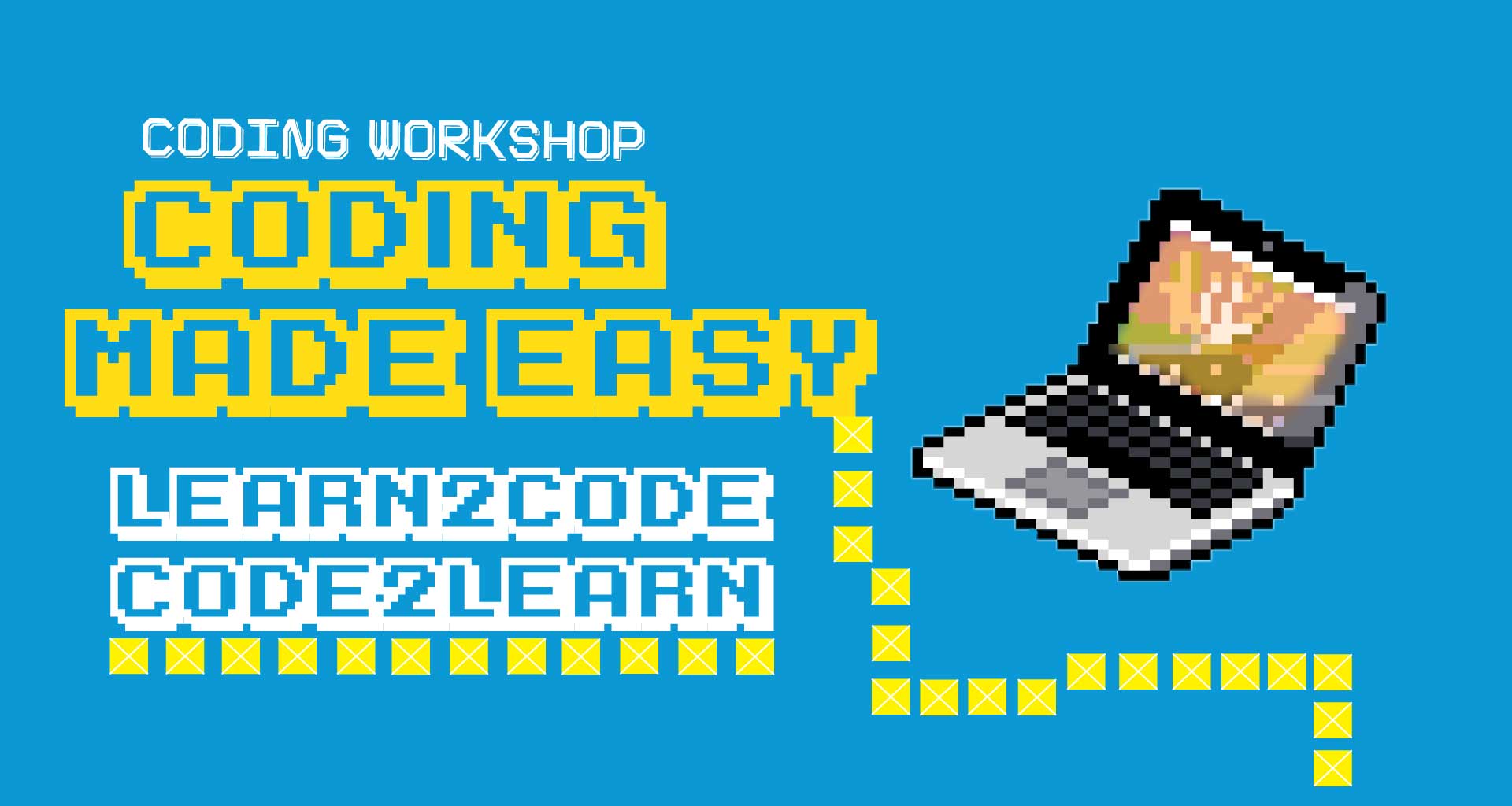
Swift is a powerful and intuitive programming language developed by Apple for building iOS, macOS, watchOS, and tvOS applications. It was first introduced in 2014 and has since become one of the most popular programming languages in the world. With its modern design, Swift makes it easy for developers to create high-performance, modern apps with a clean and easy-to-read syntax. In this article, we will explore the world of Swift coding, covering its basics, advanced features, and best practices for coding like a pro.
Introduction to Swift Basics

Before diving into the world of Swift coding, it’s essential to understand the basics of the language. Swift is an object-oriented language that supports protocols, classes, structures, and enumerations. It also has a strong focus on safety, with features like memory safety, type safety, and error handling. Some of the key features of Swift include its type inference, which allows developers to write code without explicitly specifying the type of every variable, and its optional types, which enable developers to handle null or missing values in a safe and efficient way.
Swift also has a protocol-oriented programming model, which allows developers to define blueprints for methods, properties, and other requirements that can be adopted by classes, structures, and enumerations. This approach provides a lot of flexibility and makes it easy to write generic code that can be reused across different parts of an app. With its extensions, developers can even add new functionality to existing types, making it easy to extend the language itself.
Variables, Constants, and Data Types
In Swift, developers can declare variables and constants using the let
and var
keywords. Variables are used to store values that can change over time, while constants are used to store values that never change. Swift also has a range of built-in data types, including integers, floating-point numbers, strings, and booleans. Developers can use these data types to create complex data structures, such as arrays, dictionaries, and sets.
For example, the following code declares a variable name
and assigns it the value “John”:
var name = “John”
Developers can also use the typealias
keyword to create custom data types that are easier to read and use. For example:
typealias Person = (name: String, age: Int) let person: Person = (name: “John”, age: 30)
Data Type | Description |
---|---|
Int | A whole number, such as 1, 2, or 3 |
Double | A decimal number, such as 3.14 or -0.5 |
String | A sequence of characters, such as "hello" or 'hello' |
Bool | A boolean value, which can be either true or false |

Control Flow and Functions

Control flow is an essential part of any programming language, and Swift provides a range of control flow statements to help developers manage the flow of their code. These include if
statements, switch
statements, for
loops, and while
loops. Developers can use these statements to make decisions, repeat tasks, and iterate over collections of data.
Functions are another critical part of Swift programming, and they allow developers to encapsulate blocks of code that can be reused throughout an app. Functions can take parameters and return values, making them a powerful tool for organizing and structuring code. In Swift, functions are declared using the func
keyword, and they can be called using the function name followed by parentheses containing the required parameters.
For example, the following code declares a function greet
that takes a name
parameter and prints a personalized greeting:
func greet(name: String) { print(“Hello, (name)!”) }
Developers can call this function by passing a string value, such as “John”:
greet(name: “John”)
Object-Oriented Programming
Swift is an object-oriented language, and it provides a range of features to support object-oriented programming. These include classes, structures, and enumerations, which can be used to create custom data types that encapsulate data and behavior. Classes are reference types, while structures and enumerations are value types.
Developers can use inheritance to create a hierarchy of classes, where a subclass inherits the properties and methods of its superclass. They can also use polymorphism to write code that can work with different types of objects, without knowing the specific type at compile time.
For example, the following code declares a class Person
with a name
property and a greet
method:
class Person { let name: Stringinit(name: String) { self.name = name } func greet() { print("Hello, my name is name)!") }
}
Developers can create a subclass Student
that inherits from Person
and adds a major
property:
class Student: Person { let major: Stringinit(name: String, major: String) { self.major = major super.init(name: name) } func declareMajor() { print("My major is major)") }
}
What is the difference between a class and a structure in Swift?
+In Swift, a class is a reference type, while a structure is a value type. This means that when you assign a class instance to a new variable, both variables will refer to the same instance. When you assign a structure instance to a new variable, a copy of the instance will be created.
Memory Management and Performance

Memory management is an essential part of any programming language, and Swift provides a range of features to help developers manage memory effectively. These include automatic reference counting (ARC), which automatically manages the memory used by objects, and manual memory management using pointers.
Developers can use weak references to prevent retain cycles, where two or more objects hold strong references to each other, preventing them from being deallocated. They can also use unowned references to create references that are not retained, but are still valid as long as the referenced object exists.
For example, the following code declares a class Person
with a name
property and a friend
property that uses a weak reference:
class Person { let name: String weak var friend: Person?init(name: String) { self.name = name }
}
Developers can create two Person
instances and set their friend
properties to each other:
let person1 = Person(name: “John”) let person2 = Person(name: “Jane”) person1.friend = person2 person2.friend = person1
Concurrency and Parallelism
Concurrency and parallelism are critical features in modern programming languages, and Swift provides a range of features to support concurrent and parallel programming. These include Grand Central Dispatch (GCD), which provides a high-level API for concurrent programming, and OperationQueue, which provides a high-level API for parallel programming.
Developers can use GCD to execute tasks concurrently on multiple threads, improving the responsiveness and performance of their apps. They can also use OperationQueue to execute tasks in parallel, taking advantage of multiple CPU cores to improve performance.
For example, the following code uses GCD to execute a task concurrently on a background thread:
DispatchQueue.global(qos: .background).async { // Execute a task in the background print(“Task executed in the background”) }
In conclusion, Swift is a powerful and intuitive programming language that makes it easy for developers to create high-performance, modern apps. With its modern design, Swift provides a range of features to support object-oriented programming, memory management, and concurrency. By following best practices and using the latest features and technologies, developers can create amazing apps that delight and engage users.